In this chapter we’ll build, test, and deploy a Pages app that has a homepage and an about page. We’ll also learn about Django’s class-based views and templates which are the building blocks for the more complex web applications built later on in the book.
- Chapter 2: Patterns And Relationsmr. Mac's Page Numbering
- Chapter 2: Patterns And Relationsmr. Mac's Page Printable
Initial Setup
- Textbook: McGraw-Hill My Math Grade 5 Volume 1 ISBN: 243. Use the table below to find videos, mobile apps, worksheets and lessons that supplement McGraw-Hill My Math Grade 5 Volume 1 book.
- MATH TEST- Friday, September 25- My kids will have their first math test in grade 3. Chapter 1- Patterns in Mathematics The test will be very similar to the workbook that we do in class and their homework book that they have been working on at home for homework.
- Copy and paste code samples, organize your favorites, down- load chapter s, bookmark key sections, create notes, print out pages, and benefit from tons of other time-saving features. 278 pages, published by mrbankim.debnath, 2014-02-12 07:01:49.
The pituitary accomplishes the same pattern. Positive feedback also occurs in behavior, economics, and house fires. In positive feedback the response grows and grows until it crashes. Example; Behavior: think of a concert and an encore. We saw Fleetwood Mac in N. Little Rock a few years ago. In this chapter we’ll build, test, and deploy a Pages app that has a homepage and an about page. We’ll also learn about Django’s class-based views and templates which are the building blocks for the more complex web applications built later on in the book. As in Chapter 2, our initial set up involves the following steps.
As in Chapter 2, our initial set up involves the following steps:
- create a directory for our code
- install Django in a new virtual environment
- create a new Django project
- create a new
pages
app - update
config/settings.py
On the command line make sure you’re not working in an existing virtual environment. If there is text before the dollar sign ($
) in parentheses, then you are! Make sure to type exit
to leave it.
We will again create a new directory called pages
for our project on the Desktop, but, truthfully you can put your code anywhere you like on your computer. It just needs to be in its own directory that is easily accessible.
Within a new command line console start by typing the following:
Open your text editor and navigate to the file config/settings.py
. Add the pages
app at the bottom of the INSTALLED_APPS
setting:
Start the local web server with runserver
.
And then navigate to http://127.0.0.1:8000/
.
Templates
Every web framework needs a convenient way to generate HTML files and in Django the approach is to use templates: individual HTML files that can be linked together and also include basic logic.
Recall that in the previous chapter our “Hello, World” site had the phrase hardcoded into a views.py
file as a string. That technically works but doesn’t scale well! A better approach is to link a view to a template, thereby separating the information contained in each.
In this chapter we’ll learn how to use templates to more easily create our desired homepage and about page. And in future chapters, the use of templates will support building websites that can support hundreds, thousands, or even millions of webpages with a minimal amount of code.
The first consideration is where to place templates within the structure of a Django project. There are two options. By default, Django’s template loader will look within each app for related templates. However the structure is somewhat confusing: each app needs a new templates
directory, another directory with the same name as the app, and then the template file.
Therefore, in our pages
app, Django would expect the following layout:
This means we would need to create a new templates
directory, a new directory with the name of the app, pages
, and finally our template itself which is home.html
.
Why this seemingly repetitive approach? The short answer is that the Django template loader wants to be really sure it finds the correct template! What happens if there are home.html
files within two separate apps? This structure makes sure there are no such conflicts.
There is, however, another approach which is to instead create a single project-level templates
directory and place all templates within there. By making a small tweak to our config/settings.py
file we can tell Django to also look in this directory for templates. That is the approach we’ll use.
First, quit the running server with the Control+c
command. Then create a directory called templates
and an HTML file called home.html
.
Next we need to update config/settings.py
to tell Django the location of our new templates
directory. This is a one-line change to the setting 'DIRS'
under TEMPLATES
.
Then we can add a simple headline to our home.html
file.
Ok, our template is complete! The next step is to configure our url and view files.
Class-Based Views
Early versions of Django only shipped with function-based views, but developers soon found themselves repeating the same patterns over and over again. Write a view that lists all objects in a model. Write a view that displays only one detailed item from a model. And so on.
Function-based generic views were introduced to abstract these patterns and streamline development of common patterns. However, there was no easy way to extend or customize these views. As a result, Django introduced class-based generic views that make it easy to use and also extend views covering common use cases.
Classes are a fundamental part of Python but a thorough discussion of them is beyond the scope of this book. If you need an introduction or refresher, I suggest reviewing the official Python docs which have an excellent tutorial on classes and their usage.
In our view we’ll use the built-in TemplateView to display our template. Update the pages/views.py
file.
Note that we’ve capitalized our view, HomePageView
, since it’s now a Python class. Classes, unlike functions, should always be capitalized. The TemplateView
already contains all the logic needed to display our template, we just need to specify the template’s name.
URLs
The last step is to update our URLConfs. Recall from Chapter 2 that we need to make updates in two locations. First, we update the config/urls.py
file to point at our pages
app and then within pages
we match views to URL routes.
Let’s start with the config/urls.py
file.
The code here should be review at this point. We add include
on the second line to point the existing URL to the pages
app. Next create an app-level urls.py
file.
And add the following code.
This pattern is almost identical to what we did in Chapter 2 with one major difference: when using Class-Based Views, you always add as_view()
at the end of the view name.
And we’re done! If you start up the web server with python manage.py runserver
and navigate to http://127.0.0.1:8000/
you can see our new homepage.
Add an About Page
The process for adding an about page is very similar to what we just did. We’ll create a new template file, a new view, and a new url route.
Quit the server with Control+c
and create a new template called about.html
.
Then populate it with a short HTML headline.
Create a new view for the page.
And then connect it to a url at about/
.
Start up the web server with python manage.py runserver
, navigate to http://127.0.0.1:8000/about, and you can see our new “About page”.
Extending Templates
The real power of templates is their ability to be extended. If you think about most websites, there is content that is repeated on every page (header, footer, etc). Wouldn’t it be nice if we, as developers, could have one canonical place for our header code that would be inherited by all other templates?
Well we can! Let’s create a base.html
file containing a header with links to our two pages. Type Control+c
and then create the new file.
Django has a minimal templating language for adding links and basic logic in our templates. You can see the full list of built-in template tags here in the official docs. Template tags take the form of {%something%}
where the “something” is the template tag itself. You can even create your own custom template tags, though we won’t do that in this book.
To add URL links in our project we can use the built-in url template tag which takes the URL pattern name as an argument. Remember how we added optional URL names to our two routes in pages/urls.py
? This is why. The url
tag uses these names to automatically create links for us.
The URL route for our homepage is called home
therefore to configure a link to it we would use the following: {%url'home'%}
.
At the bottom we’ve added a block
tag called content
. Blocks can be overwritten by child templates via inheritance. While it’s optional to name our closing endblock
–you can just write {%endblock%}
if you prefer–doing so helps with readability, especially in larger template files.
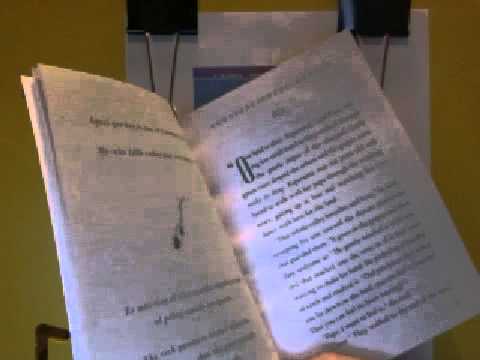
Let’s update our home.html
and about.html
files to extend the base.html
template. That means we can reuse the same code from one template in another template. The Django templating language comes with an extends method that we can use for this.
Now if you start up the server with python manage.py runserver
and open up our web pages again at http://127.0.0.1:8000/
and http://127.0.0.1:8000/about
you’ll see the header is magically included in both locations.
Nice, right?
There’s a lot more we can do with templates and in practice you’ll typically create a base.html
file and then addadditional templates on top of it in a robust Django project. We’ll do this later on in the book.
Tests
Finally we come to tests. Even in an application this basic, it’s important to add tests and get in the habit of always adding them to our Django projects. In the words of Jacob Kaplan-Moss, one of Django’s original creators, “Code without tests is broken as designed.”
Writing tests is important because it automates the process of confirming that the code works as expected. In an app like this one, we can manually look and see that the home page and about page exist and contain the intended content. But as a Django project grows in size there can be hundreds if not thousands of individual web pages and the idea of manually going through each page is not possible. Further, whenever we make changes to the code–adding new features, updating existing ones, deleting unused areas of the site–we want to be sure that we have not inadvertently broken some other piece of the site. Automated tests let us write one time how we expect a specific piece of our project to behave and then let the computer do the checking for us.
And fortunately, Django comes with built-intesting tools for writing and running tests.
If you look within our pages
app, Django already provided a tests.py
file we can use. Open it and add the following code:
We’re using SimpleTestCasehere since we aren’t using a database. If we were using a database, we’d instead useTestCase. Then we perform a check if the status code for each page is 200, which is thestandard response for a successful HTTP request. That’s a fancy way of saying it ensures that a given webpage actually exists, but says nothing about the content of said page.
To run the tests quit the server Control+c
and type python manage.py test
on the command line:
Chapter 2: Patterns And Relationsmr. Mac's Page Numbering
Success! We’ll do much more with testing in the future, especially once we start working with databases. For now, it’simportant to see how easy it is to add tests each and every time we add new functionality to our Django project.
Git and GitHub
It’s time to track our changes with git and push them up to GitHub. We’ll start by initializing our directory.
Use git status
to see all our code changes then git add -A
to add them all. Finally we’ll add our first commit message.
Over on GitHub create a new repo. Its repository name will be pages-app
and make sure to select the “Private” radio button and then click on the “Create repository” button.
On the next page scroll down to where it says “…or push an existing repository from the command line.” Copy and paste the two commands there into your terminal.
It should look like the below albeit instead of wsvincent
as the username it will be your GitHub username.
Local vs Production
To make our site available on the Internet where everyone can see it, we need to deploy our code to an external server and database. This is called putting our code into production. Local code lives only on our computer; production code lives on an external server available to everyone.
Chapter 2: Patterns And Relationsmr. Mac's Page Printable
The startproject
command creates a new project configured for local development via the file config/settings.py
. This ease-of-use means when it does come time to push the project into production, a number of settings have to be changed.
One of these is the web server. Django comes with its own basic server, suitable for local usage, but it is not suitable for production. There are two options available: Gunicorn and uWSGI. Gunicorn is the simpler to configure and more than adequate for our projects so that will be what we use.
For our hosting provider we will use Heroku because it is free for small projects, widely-used, and has a relatively straightforward deployment process.
Heroku
You can sign up for a free Heroku account on their website. After you confirm your email Heroku will redirect you to the dashboard section of the site.
Now we need to install Heroku’s Command Line Interface (CLI) so we can deploy from the command line. We want to install Heroku globally so it is available across our entire computer. Open up a new command line tab: Command+t
on a Mac, Control+t
on Windows. If we installed Heroku within our virtual environment, it would only be available there.
Within this new tab, on a Mac use Homebrew to install Heroku:
On Windows, see the Heroku CLI page to correctly install either the 32-bit or 64-bit version. If you are using Linux there are specific install instructions available on the Heroku website.
Once installation is complete you can close our new command line tab and return to the initial tab with the pages
virtual environment active.
Type the command heroku login
and use the email and password for Heroku you just set.
Deployment typically requires a number of discrete steps. It is common to have a “checklist” for these since there quickly become too many to remember. At this stage, we are intentionally keeping things basic so there are only two additional steps required, however this list will grow in future projects as we add additional security and performance features.
Here is the deployment checklist:
- install
Gunicorn
- add a
Procfile
file - update
ALLOWED_HOSTS
Gunicorn
can be installed using Pipenv.
The Procfile
is a Heroku-specific file that can include multiple lines but, in our case, will have just one specifying the use of Gunicorn as the production web server. Create the Procfile
now.
Open the Procfile
with your text editor and add the following line.
The ALLOWED_HOSTS setting represents which host/domain names our Django site can serve. This is a security measure to prevent HTTP Host header attacks, which are possible even under many seemingly-safe web server configurations. For now, we’ll use the wildcard asterisk, *
, which means all domains are acceptable. Later in the book we’ll see how to explicitly list the domains that should be allowed.
Within the config/settings.py
, scroll down to ALLOWED_HOSTS
and add a '*'
so it looks as follows:
{title=”Code”,lang=”python”}
That’s it. Keep in mind we’ve taken a number of security shortcuts here but the goal is to push our project into production in as few steps as possible.
Use git status
to check our changes, add the new files, and then commit them:
Finally push to GitHub so we have an online backup of our code changes.
Deployment
The last step is to actually deploy with Heroku. If you’ve ever configured a server yourself in the past, you’ll be amazed at how much simpler the process is with a platform-as-a-service provider like Heroku.
Our process will be as follows:
- create a new app on Heroku
- disable the collection of static files (we’ll cover this in a later chapter)
- push the code up to Heroku
- start the Heroku server so the app is live
- visit the app on Heroku’s provided URL
We can do the first step, creating a new Heroku app, from the command line with heroku create
. Heroku will create a random name for our app, in my case fathomless-hamlet-26076
. Your name will be different.
We only need to do one set of Heroku configurations at this point, which is to tell Heroku to ignore static files like CSS and JavaScript which Django by default tries to optimize for us. We’ll cover this in later chapters so for now just run the following command.
Now we can push our code to Heroku.
If we just typed git push origin master
, then the code would have been pushed to GitHub, not Heroku. Adding heroku
to the command sends the code to Heroku. This is a little confusing the first few times.
Finally, we need to make our Heroku app live. As websites grow in traffic they need additional Heroku services but for our basic example we can use the lowest level, web=1
, which also happens to be free!
Type the following command.
We’re done! The last step is to confirm our app is live and online. If you use the command heroku open
your web browser will open a new tab with the URL of your app:
Mine is at https://fathomless-hamlet-26076.herokuapp.com/. You can see the homepage is up:
You do not have to log out or exit from your Heroku app. It will continue running at this free tier on its own, though you should type exit
to leave the local virtual environment and be ready for the next chapter.
Conclusion
Congratulations on building and deploying your second Django project! This time we used templates, class-based views, explored URLConfs more fully, added basic tests, and used Heroku. Next up we’ll move on to our first database-backed project, a Message Board website, and see where Django really shines.
This concludes the free sample chapters of the book. There are 13 more…
The complete book features 16 chapters and goes in-depth on how to build both a blog app with user authentication and a newspaper site with articles and comments. Additional concepts covered include custom user models, Bootstrap for styling, password change and reset, sending email, permissions, foreign keys, and more.
